Are you looking for a VBA macro that will tell you what names start with a given letter? We came up with one for you!
This macro will prompt you for a letter and then share a list of boy names and a separate list of girl names with that letter. Here is a screenshot of it asking for the letter:
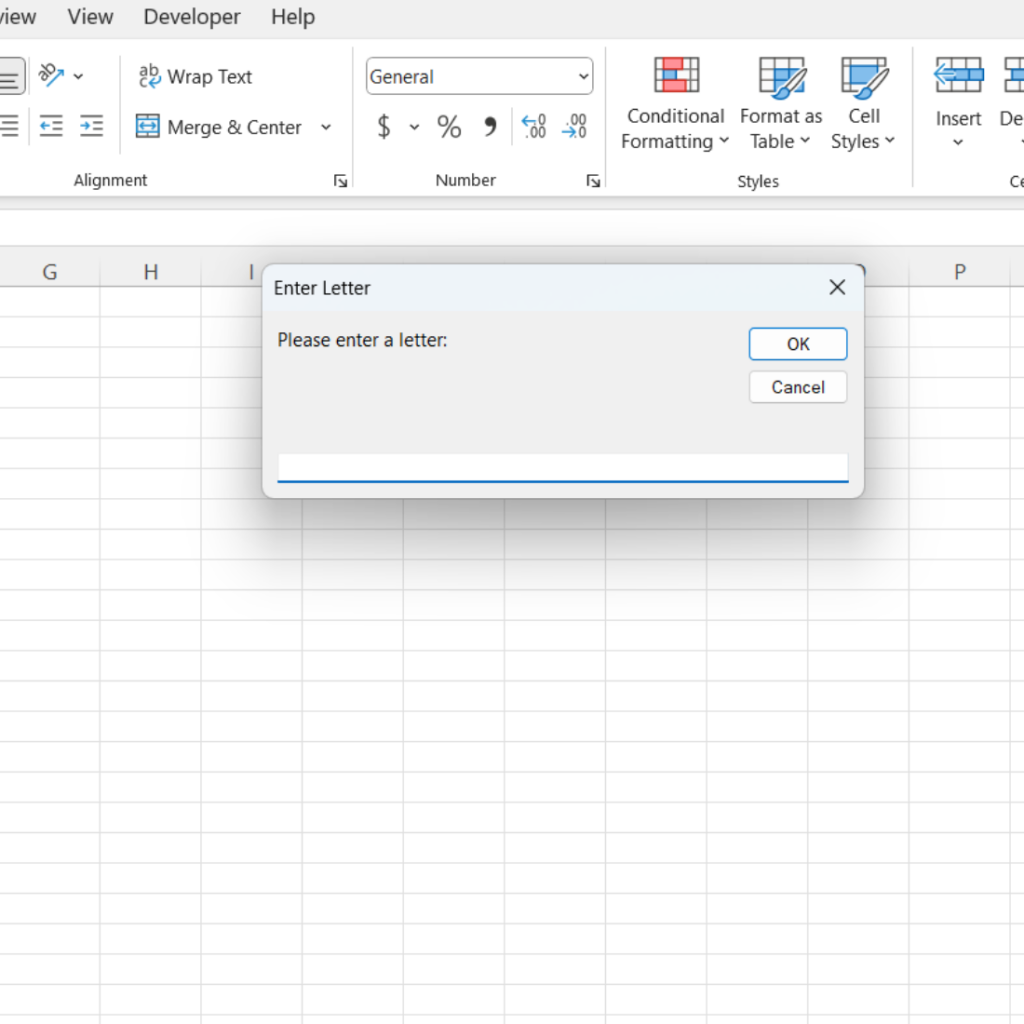
And here is the output after you enter the letter “a”:
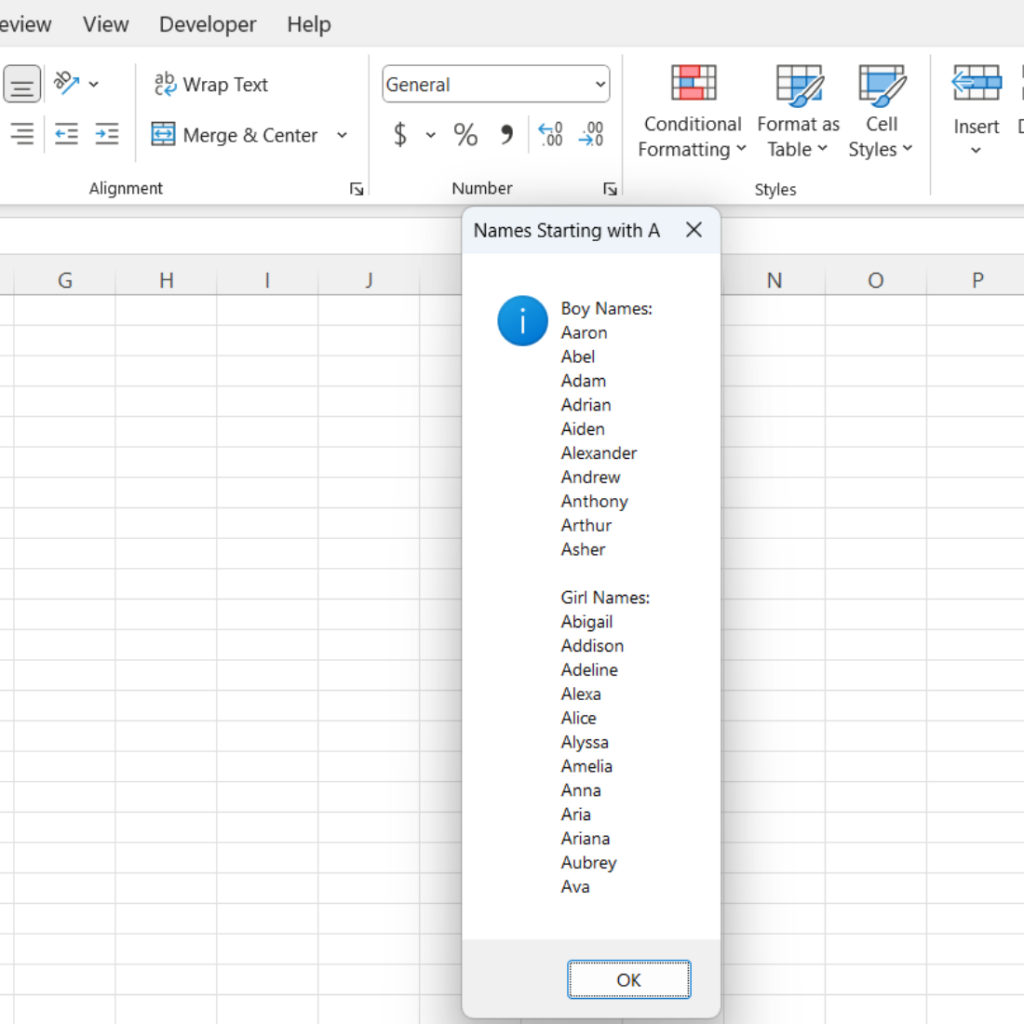
The code is listed below. You can obviously add more names to the array. I tried adding the 1,000 most common boy names and 1,000 most common girl names. However, it is a tedious process to add them all at once, because you get an error that there are too many line continuations if you try to copy and paste them in the array at one time. However, if you are ambitious, you can create a very large list with them all.
Sub ShowNamesByLetter()
‘ Define arrays for boy and girl names
Dim boyNames As Variant
Dim girlNames As Variant
boyNames = Array("Aaron", "Abel", "Adam", "Adrian", "Aiden", "Alexander", "Andrew", "Anthony", "Arthur", "Asher", _
"Benjamin", "Blake", "Brandon", "Brian", "Bryce", "Caleb", "Cameron", "Carter", "Charles", "Christian", _
"Christopher", "Connor", "Daniel", "David", "Dominic", "Dylan", "Elijah", "Elliot", "Ethan", "Evan", _
"Gabriel", "Gavin", "George", "Grayson", "Henry", "Hudson", "Hunter", "Isaac", "Isaiah", "Jack", _
"Jackson", "Jacob", "James", "Jayden", "John", "Jonathan", "Joseph", "Joshua", "Julian", "Justin", _
"Kevin", "Landon", "Liam", "Logan", "Lucas", "Luke", "Mason", "Matthew", "Michael", "Nathan", _
"Nathaniel", "Nicholas", "Noah", "Oliver", "Owen", "Patrick", "Paul", "Peter", "Quentin", "Ryan", _
"Samuel", "Sebastian", "Thomas", "Tyler", "William", "Wyatt", "Zachary")
girlNames = Array("Abigail", "Addison", "Adeline", "Alexa", "Alice", "Alyssa", "Amelia", "Anna", "Aria", "Ariana", _
"Aubrey", "Ava", "Bella", "Brianna", "Brooke", "Camila", "Caroline", "Charlotte", "Chloe", "Claire", _
"Clara", "Daisy", "Delilah", "Ella", "Ellie", "Emily", "Emma", "Eva", "Evelyn", "Faith", _
"Gabriella", "Grace", "Hannah", "Harper", "Hazel", "Isabella", "Isabelle", "Jasmine", "Julia", "Katherine", _
"Kayla", "Layla", "Leah", "Lillian", "Lily", "Lucy", "Madeline", "Madison", "Maya", "Mia", _
"Natalie", "Nora", "Olivia", "Penelope", "Peyton", "Quinn", "Riley", "Samantha", "Sarah", "Savannah", _
"Scarlett", "Sophia", "Sophie", "Stella", "Taylor", "Victoria", "Violet", "Zoe", "Zoey")
' Prompt user for a letter
Dim inputLetter As String
inputLetter = InputBox("Please enter a letter:", "Enter Letter")
' Check if input is a single letter
If Len(inputLetter) <> 1 Or Not inputLetter Like "[A-Za-z]" Then
MsgBox "Please enter a single letter.", vbExclamation, "Invalid Input"
Exit Sub
End If
' Convert input to uppercase for case-insensitive comparison
inputLetter = UCase(inputLetter)
' Find matching names
Dim matchingBoyNames As String
Dim matchingGirlNames As String
matchingBoyNames = ""
matchingGirlNames = ""
Dim name As Variant
For Each name In boyNames
If Left(UCase(name), 1) = inputLetter Then
matchingBoyNames = matchingBoyNames & name & vbCrLf
End If
Next name
For Each name In girlNames
If Left(UCase(name), 1) = inputLetter Then
matchingGirlNames = matchingGirlNames & name & vbCrLf
End If
Next name
' Display results
If matchingBoyNames = "" And matchingGirlNames = "" Then
MsgBox "No names found starting with the letter " & inputLetter & ".", vbInformation, "No Matches"
Else
Dim result As String
result = "Boy Names:" & vbCrLf & matchingBoyNames & vbCrLf & "Girl Names:" & vbCrLf & matchingGirlNames
MsgBox result, vbInformation, "Names Starting with " & inputLetter
End If
End Sub
We hope you enjoy this macro!